In this article we are going to create an Asp.Net MVC 5 application with React.Net lib and V8 engine to render the react component on server side rendering approach, so let’s get started.
Here I’m not going to give much explanation about react.js as there are plenty of articles are available on internet, you can go through those articles.
Below are the libraries we are going to use in this article,
- react.js
- ReactJS.NET (MVC 4 and 5)
- ReactJS.NET – ClearScript V8
Step 1
Create a new Empty ASP.NET MVC Project.

Step 2
Create one Controller in Controller folder named HomeController and click on Add as this will create a controller.
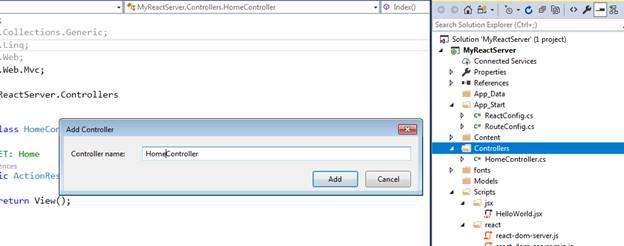
Step 3
Go to Index method and create one view called Index, this will create a master page and some required JavaScript files along with views.
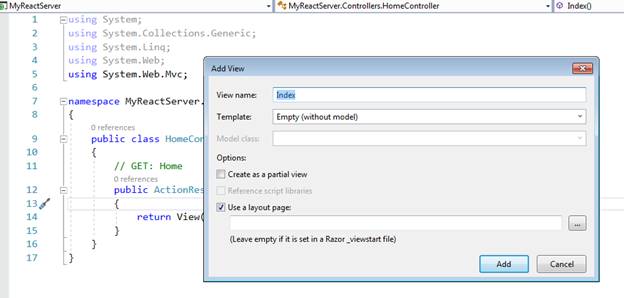
Step 4
Right click on Project and go to Nuget Package Manager and search for ReactJs.Net and install the React.Web.MVC4 package
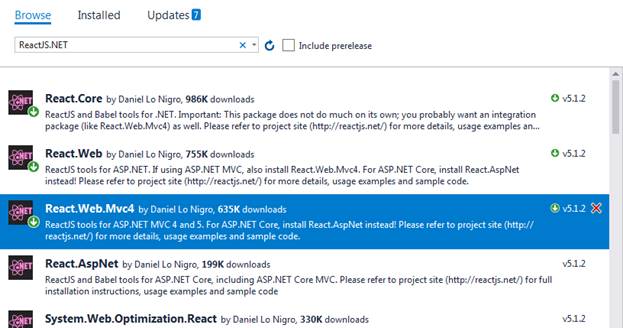
Similarly, also install the below packages from Nuget Package manager,
- React.js -- (which will install the required js library from Nuget)
- JavaScriptEngineSwitcher.V8 -- (which will install V8JsEngine` (wrapper for the Microsoft ClearScript.V8))
- JavaScriptEngineSwitcher.V8.Native.win-x86 – (please install this package based on your system type i.e. 32or 64 in my case it was 32 so I have installed x86)
Now we have installed all required packages and libraries from Nuget, so in the next step, we will get into action.
Step 5
Go to Scripts folder and create one folder called jsx where we will store all react jsx files, so inside jsx folder create one file called HelloWorld.jsx like shown below,
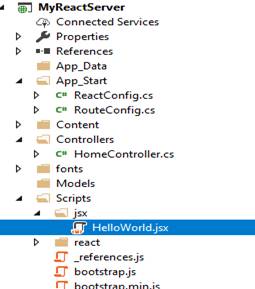
Step 6
Add the below code in the HelloWorld.jsx which will just render the hello World message in browser.
- class HelloWorld extends React.Component {
- render() {
- return (<div>
- <h1>Hello World !</h1>
- </div>);
- }
- }
- ReactDOM.render(
- <HelloWorld />,
- document.getElementById('content')
- );
As we can see in the above code we have created one class called HelloWorld and we are not importing any react or react-dom library as we are going to use server side rendering these objects are available globally.
In the next method, we are using ReactDOM.render method will render the class object in the content id.
Step 7
Go to _Layout.cshtml and add the below script to map the react js library in the master page, so please add this script at the bottom end of the body tag in layout.cshtml
@RenderSection("reactScripts",required:false)
As we are going to render the script from child page(Index.cshtml) so we have added RenderSection method.
Step 8
Open the index.csthml and add the below code as we are using RenderSection in master page and also refer the helloworld.jsx,
- @section reactScripts{
- <script src="~/Scripts/react/react.js"></script>
- <script src="~/Scripts/react/react-dom.js"></script>
- <script src="@Url.Content("~/Scripts/jsx/HelloWorld.jsx")"></script>
- }
So the complete code of index.cshtml will look like the below code, we are adding one div tag to show the output of react component
- @{
- ViewBag.Title = "Index";
- }
- <h2>Index</h2>
- <div id="content"></div>
- @section reactScripts{
- <script src="~/Scripts/react/react.js"></script>
- <script src="~/Scripts/react/react-dom.js"></script>
- <script src="@Url.Content("~/Scripts/jsx/HelloWorld.jsx")"></script>
- }
Step 9
Next step is very important as we are going to configure react component using server-side with V8JS engine, so open the ReactConfig.cs which will be auto-created when we install the ReactJs.Net and add the below code.
- ReactSiteConfiguration.Configuration
- .AddScript("~/Scripts/jsx/HelloWorld.jsx");
- JsEngineSwitcher.Current.DefaultEngineName = V8JsEngine.EngineName;
- JsEngineSwitcher.Current.EngineFactories.AddV8();
In the above code we are referring the jsx file where we have added the code to display the hello world if have any jsx files that needs to be rendered in server side needs to be added here.
In the second line we have configured the V8 Js Engine to the project.
Step 10
Call the ReactConfig.cs the method called ReactConfig.Configure() in gloabal.asax.cs file and save it.
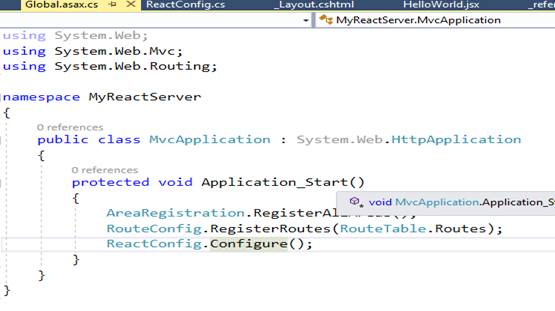
Step 11
Run it u will find the hello world in browser.
Comments
Post a Comment